This macro will create a folder and place inside of it a part document file and
3 dxf files. A user form pops up asking for box dimensions (inner measurements), thickness of the material being cut, amount of overhang to be sanded off later, and whether or not to
add handles to the shorter sides of your box. Since this macro is creating a part file you'll need to adjust line 57 of the main code (not the userForm code) to point to your version of solidWorks' Part.prtdot file. Also, take
a look at line 23 of the code. You have to have a folder named Boxes located at "C:\Solidworks Files\Boxes\". This macro creates folders inside that Boxes folder to hold your part file and 3 dxf files.

- 'Preconditions: Adjust line 29 of the code to point to the folder you wish to store all generated folders and files.
- 'Adjust line 57 to point to the solidworks default part file on your system.
- Dim swApp As SldWorks.SldWorks
- Dim part As SldWorks.ModelDoc2
- Dim boolstatus As Boolean
- Dim longstatus As Long, longwarnings As Long
- Dim thick As Long
- Dim myModelView As ModelView
- Dim final As Double
- Dim final2 As Double
- Dim sModelName As String
- Dim sPathName As String
- Dim varAlignment As Variant
- Dim dataAlignment(11) As Variant
- Dim dataAlignment2(11) As Variant
- Dim varViews As Variant
- Dim dataViews(0) As String
- Dim options As Long
- Dim test As String
- Dim partTitle As String
- Dim fix1 As Double
- Dim halfWidth As Double
- Dim folderPath As String
- Public addHandle As Boolean
- Sub main()
- folderPath = "c:\Solid Works Files\Boxes\"
- Set swApp = Application.SldWorks
- Set part = swApp.ActiveDoc
- Dim skSegment As Object
- UserForm1.Show
- End Sub
- Public Sub createBox(inputLength As Double, inputWidth As Double, inputHeight As Double, inputThickness As Double, inputSand As Double)
- UserForm1.Hide
- Dim temp As Double
- Dim fileName As String
- If inputLength > inputWidth Then 'handles go on shorter side so length must be less than width
- temp = inputLength
- inputLength = inputWidth
- inputWidth = temp
- End If
- fileName = inputWidth & "-" & inputLength & "-" & inputHeight & "-" & inputThickness
- '######################## CREATE FOLDER ############################################
- Dim fso
- Set fso = CreateObject("Scripting.FileSystemObject")
- If (fso.FolderExists(folderPath & fileName)) Then
- MsgBox "folder exists"
- Else
- MkDir folderPath & fileName
- End If
- '#################################### SAVE AS SLDPRT FILE ##############################################
- Set part = swApp.NewDocument("C:\ProgramData\SolidWorks\SOLIDWORKS 2017\templates\Part.prtdot", 0, 0, 0)
- swApp.ActivateDoc2 "Part1", False, longstatus
- longstatus = part.SaveAs3(folderPath & fileName & "\" & fileName & ".SLDPRT", 0, 2)
- partTitle = part.GetTitle
- swApp.CloseDoc partTitle
- Set part = swApp.OpenDoc6(folderPath & fileName & "\" & fileName & ".SLDPRT", 1, 0, "", longstatus, longwarnings)
- boolstatus = part.Extension.SelectByID2("Top Plane", "PLANE", 0, 0, 0, False, 0, Nothing, 0)
- part.SketchManager.InsertSketch True
- Dim instance As ISketchManager
- Set instance = part.SketchManager
- Dim Width, Length, Height, Thickness, Extra, Extra2, distance, distance2, sand As Double
- Width = (inputWidth * 0.0254)
- Length = inputLength * 0.0254
- Thickness = (inputThickness * 0.0254)
- Height = inputHeight * 0.0254
- Height = Height + (0.5 * 0.0254) + Thickness 'account for bottom of box
- sand = inputSand * 0.0254
- instance.AddToDB = True
- '######################## FRONT WIDTH ############################
- fix1 = inputWidth / inputThickness
- Extra = (inputWidth - (Int(fix1)) * inputThickness) * 0.5 * 0.0254
- If Extra / 0.0254 < 0.0001 Then
- Extra = 0
- End If
- If (Int(fix1)) Mod 2 = 0 Then 'odd #? then add half
- Extra = Extra + (0.5 * Thickness)
- Else
- End If
- distance = Thickness * 2 + Extra
- Set skSegment = part.SketchManager.CreateLine(Thickness, Thickness, 0#, distance, Thickness, 0#) 'over
- final = Width - (Thickness + Extra)
- Do While Width - (Thickness + Extra) - distance > 0.0000001 And Abs(final - distance + 2 * Thickness) > 0.0001 / 0.0254
- Set skSegment = part.SketchManager.CreateLine(distance, Thickness, 0#, distance, -sand, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(distance, -sand, 0#, (distance + Thickness), -sand, 0#) 'over
- Set skSegment = part.SketchManager.CreateLine((distance + Thickness), -sand, 0#, (distance + Thickness), Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine((distance + Thickness), Thickness, 0#, (distance + Thickness * 2), Thickness, 0#) 'over
- distance = distance + Thickness * 2
- Loop
- Set skSegment = part.SketchManager.CreateLine(distance, Thickness, 0#, distance, -sand, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(distance, -sand, 0#, (distance + Thickness), -sand, 0#) 'over
- Set skSegment = part.SketchManager.CreateLine(distance + Thickness, -sand, 0#, distance + Thickness, Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(distance + Thickness, Thickness, 0#, Width + Thickness, Thickness, 0#) 'over
- '####################### RIGHT LENGTH ##############################
- fix1 = inputLength / inputThickness
- Extra2 = (inputLength - (Int(fix1) * inputThickness)) * 0.5 * 0.0254
- If Int(fix1) Mod 2 = 0 Then 'if odd # then?'
- Extra2 = Extra2 + (0.5 * Thickness) 'even # of thicknesses
- End If
- distance2 = Thickness * 2 + Extra2
- final2 = Length - (Thickness + Extra2)
- Set skSegment = part.SketchManager.CreateLine((Width + Thickness), Thickness, 0#, (Width + Thickness), distance2, 0#) 'up
- Do While Length - (Thickness + Extra2) - distance2 > 0.0000001 And Abs(final2 - distance2 + 2 * Thickness) > 0.0001 / 0.0254
- Set skSegment = part.SketchManager.CreateLine((Width + Thickness), distance2, 0#, (Width + Thickness * 2 + sand), distance2, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine((Width + Thickness * 2 + sand), distance2, 0#, (Width + Thickness * 2 + sand), (distance2 + Thickness), 0#) 'up
- Set skSegment = part.SketchManager.CreateLine((Width + Thickness * 2 + sand), (distance2 + Thickness), 0#, (Width + Thickness), (distance2 + Thickness), 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, distance2 + Thickness, 0#, Width + Thickness, distance2 + Thickness * 2, 0#) 'up
- distance2 = distance2 + Thickness * 2
- Loop
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, distance2, 0#, Width + Thickness * 2 + sand, distance2, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness * 2 + sand, distance2, 0#, Width + Thickness * 2 + sand, distance2 + Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness * 2 + sand, distance2 + Thickness, 0#, Width + Thickness, distance2 + Thickness, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, distance2 + Thickness, 0#, Width + Thickness, Length + Thickness, 0#) 'up
- '############################################## BACK WIDTH #####################################################################
- distance = Width - Extra
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, Length + Thickness, 0#, distance, Length + Thickness, 0#) 'over
- Do While distance > 4 * Thickness + Extra
- Set skSegment = part.SketchManager.CreateLine(distance, Length + Thickness, 0#, distance, Length + Thickness * 2 + sand, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(distance, Length + Thickness * 2 + sand, 0#, distance - Thickness, Length + Thickness * 2 + sand, 0#) 'over
- Set skSegment = part.SketchManager.CreateLine(distance - Thickness, Length + Thickness * 2 + sand, 0#, distance - Thickness, Length + Thickness, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(distance - Thickness, Length + Thickness, 0#, distance - Thickness * 2, Length + Thickness, 0#) 'over
- distance = distance - Thickness * 2
- Loop
- Set skSegment = part.SketchManager.CreateLine(distance, Length + Thickness, 0#, distance, Length + Thickness * 2 + sand, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(distance, Length + Thickness * 2 + sand, 0#, distance - Thickness, Length + Thickness * 2 + sand, 0#) 'over
- Set skSegment = part.SketchManager.CreateLine(distance - Thickness, Length + Thickness * 2 + sand, 0#, distance - Thickness, Length + Thickness, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(distance - Thickness, Length + Thickness, 0#, Thickness, Length + Thickness, 0#) 'over
- '########################################### LEFT SIDE #########################################################
- distance2 = Length - Extra2
- Set skSegment = part.SketchManager.CreateLine(Thickness, Length + Thickness, 0#, Thickness, distance2, 0#) 'down
- Do While distance2 > 4 * Thickness + Extra2
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance2, 0#, -sand, distance2, 0#) 'left
- Set skSegment = part.SketchManager.CreateLine(-sand, distance2, 0#, -sand, distance2 - Thickness, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(-sand, distance2 - Thickness, 0#, Thickness, distance2 - Thickness, 0#) 'right
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance2 - Thickness, 0#, Thickness, distance2 - Thickness * 2, 0#) 'down
- distance2 = distance2 - Thickness * 2
- Loop
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance2, 0#, -sand, distance2, 0#) 'left
- Set skSegment = part.SketchManager.CreateLine(-sand, distance2, 0#, -sand, distance2 - Thickness, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(-sand, distance2 - Thickness, 0#, Thickness, distance2 - Thickness, 0#) 'right
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance2 - Thickness, 0#, Thickness, Thickness, 0#) 'down
- '################################ EXPORT TO DXF ##################################
- sModelName = folderPath & fileName & "\" & fileName & ".SLDPRT"
- sPathName = folderPath & fileName & "\" & fileName
- test = folderPath & fileName & "\" & fileName
- sPathName = sPathName + ".dxf"
- dataAlignment(0) = 0# 'this part controls geometry to origin relation
- dataAlignment(1) = 0#
- dataAlignment(2) = 0#
- dataAlignment(3) = 0#
- dataAlignment(4) = 0#
- dataAlignment(5) = 0#
- dataAlignment(6) = 0#
- dataAlignment(7) = 0#
- dataAlignment(8) = 0#
- dataAlignment(9) = 0#
- dataAlignment(10) = 0#
- dataAlignment(11) = 0#
- dataAlignment2(0) = 1#
- dataAlignment2(1) = 0#
- dataAlignment2(2) = 0#
- dataAlignment2(3) = 1#
- dataAlignment2(4) = 0#
- dataAlignment2(5) = 0#
- dataAlignment2(6) = 1#
- dataAlignment2(7) = 0#
- dataAlignment2(8) = 0#
- dataAlignment2(9) = 1#
- dataAlignment2(10) = 0#
- dataAlignment2(11) = 0#
- dataViews(0) = "*Top"
- varViews = dataViews
- sModelName = part.GetPathName
- part.ExportToDWG2 sPathName, sModelName, 3, True, dataAlignment, True, True, 0, varViews
- test2 = test & " Bottom.dxf"
- test = test & ".dxf"
- Name test As test2
- part.SketchManager.InsertSketch True
- '####################### FRONT WIDTH & RIGHT SIDE 1 ######################
- fix1 = (Height / 0.0254) / inputThickness
- Extra3 = ((Height / 0.0254) - (Int(fix1) * inputThickness)) * 0.5 * 0.0254
- If Int(fix1) Mod 2 = 0 Then 'if even # then?'
- Extra3 = Extra3 + (0.5 * Thickness) 'even # of thicknesses
- End If
- boolstatus = part.Extension.SelectByID2("Front Plane", "PLANE", 0, 0, 0, False, 0, Nothing, 0)
- part.SketchManager.InsertSketch True
- distance = Thickness + Extra3
- Set skSegment = part.SketchManager.CreateLine(Thickness, 0#, 0#, Width + Thickness, 0#, 0#) 'over
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, 0#, 0#, Width + Thickness, distance, 0#) 'up
- final = Height - (Thickness + Extra3) + (0.5 * 0.0254)
- Do While Height - (2 * Thickness + Extra3) - distance > 0.0000001 And Abs(final - distance + 2 * Thickness) > 0.0001 / 0.0254
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, distance, 0#, Width + Thickness * 2 + sand, distance, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness * 2 + sand, distance, 0#, Width + Thickness * 2 + sand, distance + Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness * 2 + sand, distance + Thickness, 0#, Width + Thickness, distance + Thickness, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, distance + Thickness, 0#, Width + Thickness, distance + Thickness * 2, 0#) 'up
- distance = distance + Thickness * 2
- Loop
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, distance, 0#, Width + Thickness * 2 + sand, distance, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness * 2 + sand, distance, 0#, Width + Thickness * 2 + sand, distance + Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness * 2 + sand, distance + Thickness, 0#, Width + Thickness, distance + Thickness, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, distance + Thickness, 0#, Width + Thickness, Height, 0#) 'up
- '######################################## BACK WIDTH & LEFT SIDE 1 ######################################
- Set skSegment = part.SketchManager.CreateLine(Width + Thickness, Height, 0#, Thickness, Height, 0#) 'in far/ BACK WIDTH
- distance = distance + Thickness
- Set skSegment = part.SketchManager.CreateLine(Thickness, Height, 0#, Thickness, distance, 0#) 'down
- Do While (distance) > 3 * Thickness + Extra3
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance, 0#, -sand, distance, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(-sand, distance, 0#, -sand, distance - Thickness, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(-sand, distance - Thickness, 0#, Thickness, distance - Thickness, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance - Thickness, 0#, Thickness, distance - Thickness * 2, 0#) 'down
- distance = distance - Thickness * 2
- Loop
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance, 0#, -sand, distance, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(-sand, distance, 0#, -sand, distance - Thickness, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(-sand, distance - Thickness, 0#, Thickness, distance - Thickness, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance - Thickness, 0#, Thickness, 0#, 0#) 'down
- '############################################# SQUARES 1 ##################################################
- final = Width - (Thickness + Extra)
- distance = Thickness * 2 + Extra
- Do While Width - (Extra) - distance > 0.0000001 And Abs(final - distance + 2 * Thickness) > 0.0001 / 0.0254
- Set skSegment = part.SketchManager.CreateLine(distance, 0.5 * 0.0254, 0#, distance, 0.5 * 0.0254 + Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(distance, 0.5 * 0.0254 + Thickness, 0#, distance + Thickness, 0.5 * 0.0254 + Thickness, 0#) 'over
- Set skSegment = part.SketchManager.CreateLine(distance + Thickness, 0.5 * 0.0254 + Thickness, 0#, distance + Thickness, 0.5 * 0.0254, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(distance + Thickness, 0.5 * 0.0254, 0#, distance, 0.5 * 0.0254, 0#) 'over
- distance = distance + Thickness * 2
- Loop
- '##################################### EXPORT TO DXF #####################################
- dataViews(0) = "*Front"
- varViews = dataViews
- part.ExportToDWG2 sPathName, sModelName, 3, True, dataAlignment2, True, False, 0, varViews
- test = folderPath & fileName & "\" & fileName
- test2 = test & " Side1.dxf"
- test = test & ".dxf"
- Name test As test2
- part.SketchManager.InsertSketch True
- '##################### FRONT WIDTH & RIGHT SIDE 2 #######################
- fix1 = (Height / 0.0254) / inputThickness
- Extra = ((Height / 0.0254) - (Int(fix1) * inputThickness)) * 0.5 * 0.0254
- If Int(fix1) Mod 2 = 0 Then 'if even # then?'
- Extra = Extra + (0.5 * Thickness) 'even # of thicknesses
- End If
- boolstatus = part.Extension.SelectByID2("Right Plane", "PLANE", 0, 0, 0, False, 0, Nothing, 0)
- part.SketchManager.InsertSketch True
- distance = Thickness + Extra
- Set skSegment = part.SketchManager.CreateLine(-sand, 0#, 0#, Length + Thickness * 2 + sand, 0#, 0#) 'over
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness * 2 + sand, 0#, 0#, Length + Thickness * 2 + sand, distance, 0#) 'up
- final = Height - (Thickness + Extra) + (0.5 * 0.0254)
- Do While Height - (2 * Thickness + Extra) - distance > 0.0000001 And Abs(final - distance + 2 * Thickness) > 0.0001 / 0.0254
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness * 2 + sand, distance, 0#, Length + Thickness, distance, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness, distance, 0#, Length + Thickness, distance + Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness, distance + Thickness, 0#, Length + Thickness * 2 + sand, distance + Thickness, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness * 2 + sand, distance + Thickness, 0#, Length + Thickness * 2 + sand, distance + Thickness * 2, 0#) 'up
- distance = distance + Thickness * 2
- Loop
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness * 2 + sand, distance, 0#, Length + Thickness, distance, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness, distance, 0#, Length + Thickness, distance + Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness, distance + Thickness, 0#, Length + Thickness * 2 + sand, distance + Thickness, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness * 2 + sand, distance + Thickness, 0#, Length + Thickness * 2 + sand, Height, 0#) 'up
- '############################### BACK WIDTH & LEFT SIDE 2 ###############################################################################################
- Set skSegment = part.SketchManager.CreateLine(Length + Thickness * 2 + sand, Height, 0#, -sand, Height, 0#) 'in far/ BACK WIDTH
- distance = distance + Thickness
- Set skSegment = part.SketchManager.CreateLine(-sand, Height, 0#, -sand, distance, 0#) 'down
- Do While (distance) > 3 * Thickness + Extra
- Set skSegment = part.SketchManager.CreateLine(-sand, distance, 0#, Thickness, distance, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance, 0#, Thickness, distance - Thickness, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance - Thickness, 0#, -sand, distance - Thickness, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(-sand, distance - Thickness, 0#, -sand, distance - Thickness * 2, 0#) 'down
- distance = distance - Thickness * 2
- Loop
- Set skSegment = part.SketchManager.CreateLine(-sand, distance, 0#, Thickness, distance, 0#) 'in
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance, 0#, Thickness, distance - Thickness, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(Thickness, distance - Thickness, 0#, -sand, distance - Thickness, 0#) 'out
- Set skSegment = part.SketchManager.CreateLine(-sand, distance - Thickness, 0#, -sand, 0#, 0#) 'down
- '############################################# SQUARES 2 ##########################################################
- distance = Thickness * 2 + Extra2
- Do While (Length - (Extra2) - distance) > 0.00000254 And Abs(final2 - distance + 2 * Thickness) > (0.0001 * 0.0254)
- Set skSegment = part.SketchManager.CreateLine(distance, 0.5 * 0.0254, 0#, distance, 0.5 * 0.0254 + Thickness, 0#) 'up
- Set skSegment = part.SketchManager.CreateLine(distance, 0.5 * 0.0254 + Thickness, 0#, distance + Thickness, 0.5 * 0.0254 + Thickness, 0#) 'over
- Set skSegment = part.SketchManager.CreateLine(distance + Thickness, 0.5 * 0.0254 + Thickness, 0#, distance + Thickness, 0.5 * 0.0254, 0#) 'down
- Set skSegment = part.SketchManager.CreateLine(distance + Thickness, 0.5 * 0.0254, 0#, distance, 0.5 * 0.0254, 0#) 'over
- distance = distance + Thickness * 2
- Loop
- '############################################# HANDLE #######################################################################################################
- If addHandle = True And Length >= 5 * 0.0254 And inputHeight >= 3 * 0.0254 Then
- Set skSegment = part.SketchManager.CreateArc(0.5 * Length + Thickness, Height - (1.31 * 0.0254) + 0.0762, 0#, 0.5 * Length + Thickness + 0.041651, Height - (1.31 * 0.0254) - 0.007103, 0#, 0.5 * Length + Thickness - 0.041651, Height - (1.31 * 0.0254) - 0.007103, 0#, -1) 'lower arc
- part.ClearSelection2 True
- Set skSegment = part.SketchManager.CreateArc(0.5 * Length + Thickness - 0.0381, Height - (1.31 * 0.0254), 0#, 0.5 * Length + Thickness - 0.041651, Height - (1.31 * 0.0254) - 0.007103, 0#, 0.5 * Length + Thickness - 0.034549, Height - (1.31 * 0.0254) + 0.007103, 0#, -1) 'left arc
- part.ClearSelection2 True
- Set skSegment = part.SketchManager.CreateArc(0.5 * Length + Thickness, Height - (1.31 * 0.0254) + 0.0762, 0#, 0.5 * Length + Thickness - 0.034549, Height - (1.31 * 0.0254) + 0.007103, 0#, 0.5 * Length + Thickness + 0.034549, Height - (1.31 * 0.0254) + 0.007103, 0#, 1) 'upper arc
- part.ClearSelection2 True
- Set skSegment = part.SketchManager.CreateArc(0.5 * Length + Thickness + 0.0381, Height - (1.31 * 0.0254), 0#, 0.5 * Length + Thickness + 0.034549, Height - (1.31 * 0.0254) + 0.007103, 0#, 0.5 * Length + Thickness + 0.041651, Height - (1.31 * 0.0254) - 0.007103, 0#, -1) 'right arc
- Else
- End If
- '######################################## EXPORT TO DXF ##################################
- dataViews(0) = "*Right"
- varViews = dataViews
- part.ExportToDWG2 sPathName, sModelName, 3, True, dataAlignment2, True, False, 0, varViews
- test = folderPath & fileName & "\" & fileName
- test2 = test & " Side2.dxf"
- test = test & ".dxf"
- Name test As test2
- longstatus = part.SaveAs3(folderPath & fileName & "\" & fileName & ".SLDPRT", 0, 2)
- part.SketchManager.InsertSketch True
- instance.AddToDB = False
- part.ViewZoomtofit2
- End Sub
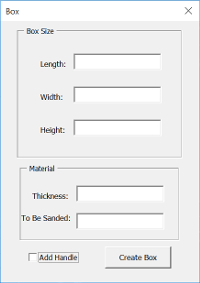
UserForm1 Code:
- Private Sub CommandButton1_Click()
- Dim boxWidth As Double
- Dim boxHeight As Double
- Dim boxLength As Double
- Dim woodThickness As Double
- Dim sand As Double
- boxWidth = textBox2.Text
- boxHeight = textBox3.Text
- boxLength = Length.Text
- woodThickness = Thickness.Text
- sand = Sanded.Text
- If CheckBox1.Value = False Then
- addHandle = False
- Else
- addHandle = True
- End If
- Call createBox(boxLength, boxWidth, boxHeight, woodThickness, sand)
- End Sub
Notes:
Because of snapping, many lines would not draw properly while this macro ran. This is no longer a problem. The solution to this issue is found on or around line 74 of the main code:
part.SketchManager.AddToDB = True
This line of code some how bypasses the snapping portion of the drawing process.
Handles will only be drawn if the height of your box is over 3 inches and the shorter of the two sides is longer than 5 inches. This is accomplished with line 302 of the main code.
In order to cut the dxf files with a laser I had to adjust the tool path inside of the laser operating software to account for the kerf (thickness) of the laser beam. This step could be avoided if the macro accounted for the laser beam's kerf.