This macro was created to quickly create washer shaped objects, but a variety of shapes are possible. With the right settings
you could quickly create rods, spheres, o-rings, square profile o-rings, spacers, toroids (donuts), and tubes. To create a sphere, leave either the inner or outer diameter box empty and set the radius to
half the diameter of the desired part. For a perfect sphere the diameter and length values will be equal while the radius value will be half the diameter value.

- 'precondition: Adjust line 23 of the code below to point to your version of solidWorks' Part.prtdot file.
- Dim swApp As Object
- Dim part As Object
- Dim boolstatus As Boolean
- Dim longstatus As Long, longwarnings As Long
- Dim swModelDocExt As SldWorks.ModelDocExtension
- Public firstEnter As Boolean
- Public firstEnter1 As Boolean
- Public firstEnter2 As Boolean
- Public firstEnter3 As Boolean
- Public firstEnter4 As Boolean
- Public isChamfer As Boolean
- Sub main()
- firstEnter = True
- firstEnter1 = True
- firstEnter2 = True
- firstEnter3 = True
- firstEnter4 = True
- Set swApp = Application.SldWorks
- Set part = swApp.NewDocument("C:\ProgramData\SolidWorks\SOLIDWORKS 2017\templates\Part.prtdot", 0, 0, 0) 'This line opens up SolidWorks' default part document. If you don't have SW 2017, or you've installed SolidWorks to a different location, this line will need to be changed.
- 'Set part = swApp.NewDocument("C:\ProgramData\SolidWorks\SOLIDWORKS 2016\templates\Part.prtdot", 0, 0, 0)
- 'Set part = swApp.NewDocument("C:\ProgramData\SolidWorks\SolidWorks 2013\templates\Part.prtdot", 0, 0, 0)
- Set part = swApp.ActiveDoc
- UserForm1.Show
- End Sub
- Sub createShape(inner, outer, length, chamfer)
- boolstatus = part.Extension.SelectByID2("Top Plane", "PLANE", 0, 0, 0, False, 0, Nothing, 0)
- part.SketchManager.InsertSketch True
- part.ClearSelection2 True
- part.SketchManager.AddToDB = True 'Without this line close diameters merge. Example: a 1 inch circle and a 1.01 inch circle snap together.
- Dim skSegment As Object
- If inner <> 0 Then
- Set skSegment = part.SketchManager.CreateCircle(0#, 0#, 0#, inner, 0#, 0#)
- End If
- If outer <> 0 Then
- Set skSegment = part.SketchManager.CreateCircle(0#, 0#, 0#, outer, 0#, 0#)
- End If
- part.SketchManager.AddToDB = False
- Dim myFeature As Object
- Set myFeature = part.FeatureManager.FeatureExtrusion2(True, False, False, 0, 0, length, chamfer, False, False, False, False, 1.74532925199433E-02, 1.74532925199433E-02, False, False, False, False, True, True, True, 0, 0, False)
- part.SelectionManager.EnableContourSelection = False
- Set swModelDocExt = part.Extension
- swModelDocExt.SelectAll
- If isChamfer Then
- Set myFeature = part.FeatureManager.InsertFeatureChamfer(6, 1, chamfer, 0.78539816339745, 0, 0, 0, 0)
- Else
- Set myFeature = part.FeatureManager.FeatureFillet3(195, chamfer, 0.01, 0, 0, 0, 0, (radiiArray0), (dist2Array0), (conicRhosArray0), (setBackArray0), (pointArray0), (pointDist2Array0), (pointRhoArray0))
- End If
- End
- End Sub
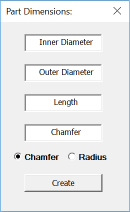
UserForm1 Code:
- Private Sub Create_Click()
- Dim inner As Double
- Dim outer As Double
- Dim length As Double
- Dim chamfer As Double
- If TextBox1.Text = "Inner Diameter" Or TextBox1.Text = "" Then
- inner = 0
- Else
- inner = TextBox1.Text * 0.0254 * 0.5
- End If
- If TextBox2.Text = "Outer Diameter" Or TextBox2.Text = "" Then
- outer = 0
- Else
- outer = TextBox2.Text * 0.0254 * 0.5
- End If
- length = TextBox3.Text * 0.0254
- If TextBox4.Text = "Chamfer" Or TextBox4.Text = "" Then
- chamfer = 0
- Else: chamfer = TextBox4.Text * 0.0254
- End If
- Call createShape(inner, outer, length, chamfer)
- End Sub
- Private Sub OptionButton1_Click()
- isChamfer = True
- If firstEnter4 Then TextBox4.Text = "Chamfer"
- End Sub
- Private Sub OptionButton2_Click()
- isChamfer = False
- If firstEnter4 Then TextBox4.Text = "Radius"
- End Sub
- Private Sub TextBox1_Enter()
- If (firstEnter = True) Then
- firstEnter = False
- TextBox1.TextAlign = fmTextAlignLeft
- TextBox1.Text = ""
- End If
- End Sub
- Private Sub TextBox2_Enter()
- If firstEnter2 Then
- TextBox2.Text = ""
- TextBox2.TextAlign = fmTextAlignLeft
- firstEnter2 = False
- End If
- End Sub
- Private Sub TextBox3_Enter()
- If firstEnter3 Then
- TextBox3.TextAlign = fmTextAlignLeft
- TextBox3.Text = ""
- firstEnter3 = False
- End If
- End Sub
- Private Sub TextBox4_Enter()
- If firstEnter4 Then
- TextBox4.TextAlign = fmTextAlignLeft
- TextBox4.Text = ""
- firstEnter4 = False
- End If
- End Sub
- Private Sub TextBox5_Enter()
- TextBox5.Visible = False
- End Sub
- Private Sub UserForm_Initialize()
- isChamfer = True
- OptionButton1.Value = True
- End Sub
Notes:
A "compile error: Expected variable or procedure, not project" was triggered when the subroutine createShape was named createWasher. A subroutine name cannot match the macro file name. CreateWasher.swp cannot have a subroutine inside of it called createWasher, so it seems.
When the value in the Inner Diameter text box was almost the same size as the value in the Outer Diameter text box, the sketch would appear to draw only one circle. Because of snapping, the second circle was never drawn. The solution to this issue is found on or around line 32 of the main code:
part.SketchManager.AddToDB = True
This line of code some how bypasses the snapping portion of the drawing process.